Customize Google Font requests
Table of contents
If you want to use a Google Font in your page, first you have to instruct the browser to download it. To do that, you have 2 choices:
You can add a <link />
tag in the <head>
of the document:
<head>
<link
href="https://fonts.googleapis.com/css?family=Montserrat"
rel="stylesheet"
/>
</head>
Or you can add a CSS import rule:
@import url("https://fonts.googleapis.com/css?family=Montserrat");
Finally, you can use it in your CSS:
body {
font-family: "Montserrat", sans-serif;
}
In both cases, you make a request to:
https://fonts.googleapis.com/css
In this URL you can add some additional parameters. You can specify:
- The font family, single or multiple. You already saw how to request a single font, the Montserrat.
- The font weight. For example, 300, 400, or 700.
- The font style. Bold, italic, or bolditalic.
- The language. Latin, Greek, Cyrillic, and many more.
- The font-display property to improve performance.
- The specific letters you want to download from a font.
- A font effect.
You can customize most of the above inside the Google Fonts app.
It’s not practical to hard code them into the URL if the app does this better. And that’s exactly what you’re going to do now. You will use the interface to customize the requests.
Choose font-family and font-display
Let’s say that you want to add fonts to your new website. You are not looking for something particular. In this case, a good choice is to use a font pairing app. So you go to the FontPair, and you pick a nice pair of fonts.
I will choose Fira Sans for the headings and Merriweather for the body.
The next step is to go to Google Fonts and select those 2 fonts. After you do that, expand the selected fonts modal at the bottom right of the screen:
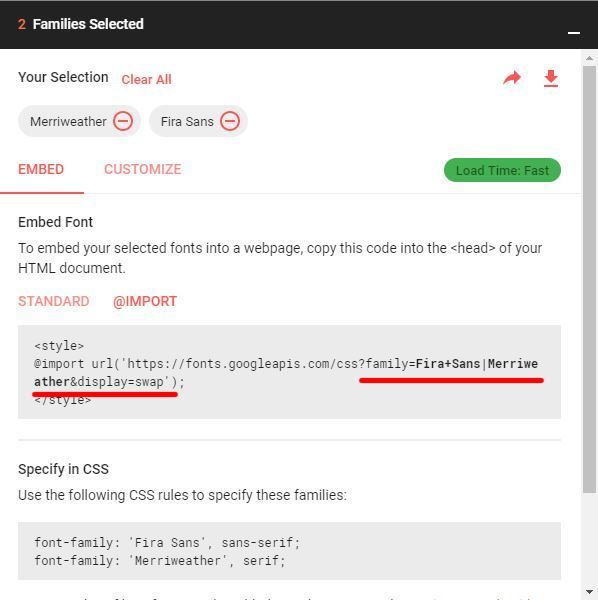
The URL Google generates for you is the following:
https://fonts.googleapis.com/css?family=Fira+Sans|Merriweather&display=swap
I can already notice 4 things from the URL above:
- To request a font family, you use the family parameter.
- If you want multiple font families, you separate them with the pipe
|
operator. - If the font name consists of multiple words, you replace the spaces with the plus operator
+
. - By default, Google sets the
font-display
parameter (display in the URL) toswap
. This means that if the font is not available in the initial page render, the browser will use the fallback fonts. This is called the Flash of Unstyled Content (or text). When the browser finally downloads the requested fonts, it will replace them. This font loading method is good for performance. You can find here more details about font loading performance.
This is fine, but you want the request to be a little more specific.
Choose font weight and font style
Because we’re using Fira Sans for the headings, it makes sense to download only the bold version. Additionally, you want the bold, the normal, and the italic version of the Merriweather font for the body.
Click on the customize tab on the selected fonts modal, and choose all the required versions. After you do that, this what the modal looks like:
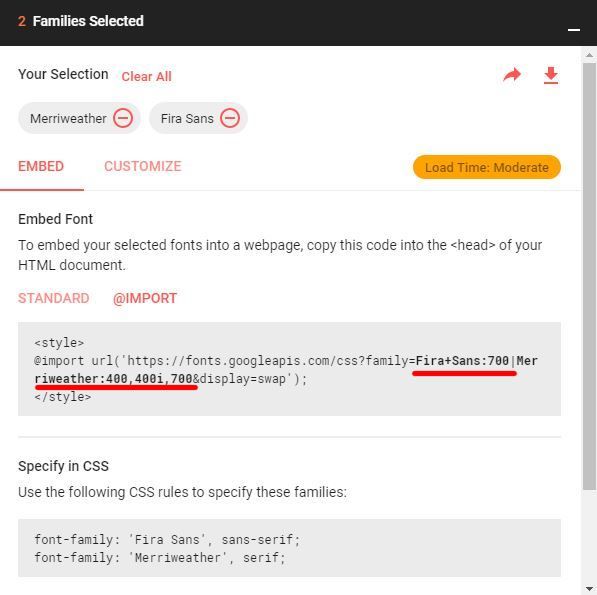
And this is the URL:
https://fonts.googleapis.com/css?family=Fira+Sans:700|Merriweather:400,400i,700&display=swap
So if you want to specify a version for a font:
- You use the colon
:
operator after the name. - If you want multiple styles, you separate them with a comma
,
. - If you want the bold version, you add 700, bold, or just b.
- If you want the normal version, you add 400 or normal.
- If you want the italic version, you add 400i, italic, or i. In the same way, if you want the bold italic, you can add 700i, bolditalic, or bi.
One more thing you can do from the UI is to choose a language. Google refers to the languages as script subsets.
Specify the language
Let’s say that you want to support English, which is the Latin subset, and Greek.
If you go back to the customize tab, and you try to select the languages, you will see that we have the following problem:
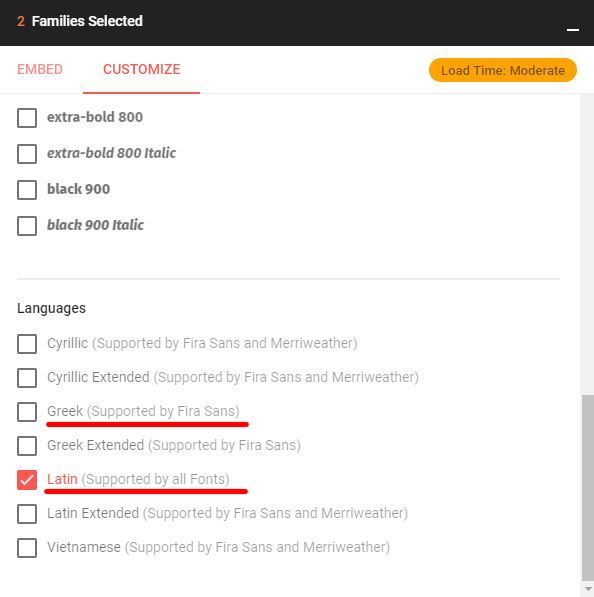
Both fonts support Latin, but Merriweather doesn’t support Greek. That means that you have to replace Merriweather with another font.
To see which fonts a language supports, you’ll have to close the selected fonts modal, and select a language as shown in the image below:
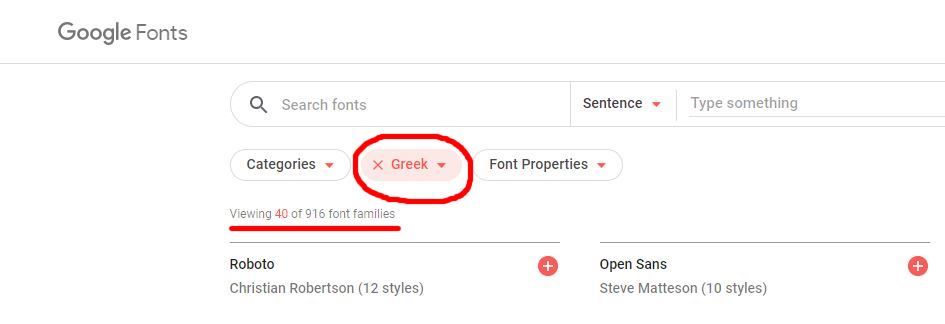
Let’s choose the Fira Mono font as a replacement for the Merriweather, and use it for the headings.
If you do that in your selected fonts modal, this is what the generated URL will look like:
https://fonts.googleapis.com/css?family=Fira+Mono:700|Fira+Sans:400,400i,700&display=swap&subset=greek
To download a subset other than Latin, you use the subset parameter. Latin is included by default, and you don’t need to specify it. In fact, in modern browsers, you don’t have to specify the subset parameter. The browser will download the correct language (if available) based on the content. You only have to check in Google Fonts UI if the font is available for the language you want to support.
You can add another performance improvement by specifying which letters you want from a font.
Download specific characters from a font
Let’s say that you want to have a different font for the logo. The logo will read “Mark’s Site” because your name is obviously Mark, not because I wrote the example earlier, and I don’t want to change it now. You figure out that a good font for the logo will be the Permanent Marker.
To specify the letters, you have to use the text parameter. But because you have to add it with an ampersand &
, you’ll have to request it in a different link tag. If you don’t, the text parameter will also apply to the previous fonts.
So your HTML now becomes:
<link
href="https://fonts.googleapis.com/css?family=Fira+Mono:700|Fira+Sans:400,400i,700&display=swap&subset=greek"
rel="stylesheet"
/>
<link
href="https://fonts.googleapis.com/css?family=Permanent+Marker&text=Mark'sSite&display=swap"
rel="stylesheet"
/>
P.S. You don’t have to make two requests; you can use the colon :
instead:
<link
href="https://fonts.googleapis.com/css?family=Fira+Mono:700|Fira+Sans:400,400i,700|Permanent+Marker:text=Mark'sSite&display=swap&subset=greek"
rel="stylesheet"
/>
Add text effects
Google also offers some ridiculous text effects. These are some pre-made CSS animations that are added in the initial CSS you download. To use them, you add a class to the element you want to style.
For example, if you want to apply the fire effect on the logo (please don’t), your logo URL changes to:
https://fonts.googleapis.com/css?family=Permanent+Marker&text=Mark'sSite&display=swap&effect=fire
and inside the HTML:
<!-- <h1 class="logo">Mark's Site!</h1> -->
<h1 class="logo font-effect-fire">Mark's Site!</h1>
Example code
This is the completed HTML file that I used in the examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1.0"
/>
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Hello, world</title>
<link
href="https://fonts.googleapis.com/css?family=Fira+Mono:700|Fira+Sans:400,400i,700&display=swap&subset=greek"
rel="stylesheet"
/>
<link
href="https://fonts.googleapis.com/css?family=Permanent+Marker&text=Mark'sSite!&display=swap&effect=fire"
rel="stylesheet"
/>
<style>
h1,
h2,
h3,
h4,
h5,
h6 {
font-family: "Fira Mono", monospace;
}
body {
font-size: 20px;
font-family: "Fira Sans", sans-serif;
}
h1.logo {
text-align: center;
font-size: 3em;
font-family: "Permanent Marker", cursive;
}
</style>
</head>
<body>
<!-- <h1 class="logo">Mark's Site!</h1> -->
<h1 class="logo font-effect-fire">Mark's Site!</h1>
<h2>English text</h2>
<p>
Lorem ipsum dolor sit, amet consectetur adipisicing elit.
Recusandae repudiandae explicabo, iste fugit asperiores laboriosam.
</p>
<i>Italic text</i>
<h2>Ελληνικό κείμενο</h2>
<p>
Το πλοίο χάθηκε στην ομίχλη τρεις ώρες έξω από το λιμάνι. Η Γη
έλαμπε σαν ημισέληνος κάτω από το ιπτάμενο σκάφος.
</p>
<i>Ιταλικό κείμενο λολ</i>
</body>
</html>
Resources
Other things to read
Popular
- Reveal animations on scroll with react-spring
- Gatsby background image example
- Extremely fast loading with Gatsby and self-hosted fonts